Face Authentication
Face Authentication is a process in which a selfie is taken and compared to a previously onboarded user to:
- Identify a user and grant access or permissions in your application.
- Get an access token to interact with a particular onboarding session.
The general process:
- Identify your users using the
renderLogin
method from the Web SDK. - Check for a face match between the user's selfie and an Incode identity.
- Validate in the backend if Incode registered the authentication attempt and was successful using the
/authentication/verify
endpoint

Pre-requisites
Before any coding, please make sure you are familiar with the following pre-requisites:
- Incode Identities
- Web SDK Standard Integration
1. Identify your users using the renderLogin()
method (Web SDK)
renderLogin()
method (Web SDK)This method renders a face recognition screen that checks if the user has an Incode Identity.
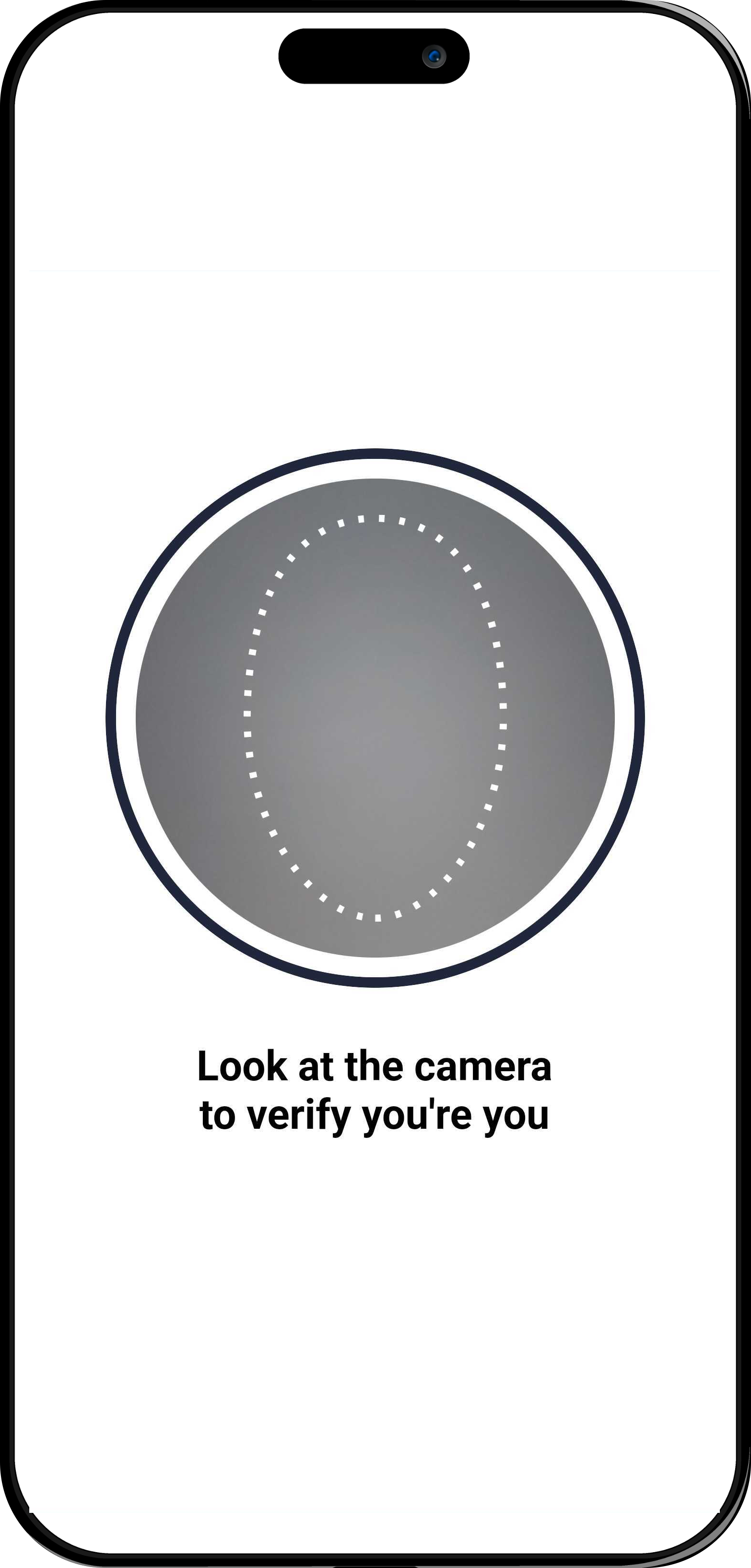
Important
Incode validates the existence of a user based on a biometric template generated at the identity creation. Please ask your Customer Success Manager for more information about confidence thresholds.
Using the renderLogin()
for Face Authentication
renderLogin()
for Face AuthenticationFirst, set an HTML with the following code, which includes the following:app.js
file.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Incode Web SDK Demo 2024</title>
<script src="https://sdk.incode.com/sdk/onBoarding-<<WEB_SDK_VERSION>>.js" defer></script>
<script src="app.js" defer></script>
</head>
<body>
<main>
<section id="incode-container"></section>
</main>
</body>
</html>
API URL for face authentication
Notice the
apiURL
ends in/0
. This path is needed so the endpoint doesn't require to start the incode SDK using the apiKey
let incode;
const container = document.querySelector("#incode-container");
Initialize the Web SDK.
function initializeIncodeSDK() {
const incode = window.OnBoarding.create({
apiURL: "<<DEMO_URL_0>>",
});
return incode;
}
Call the `renderLogin()` method to capture the user's face.
function identifyUser(){
incode.renderLogin(container,{
onSuccess: (response) => {
Check for a face match to know if the user has a registered identity.
if (response.faceMatch){
// If yes, validate the authentication (`/omni/authentication/verify`)
// You will learn how later in this guide
}
},
isOneToOne: true,
oneToOneProps: {
identityId: "The user's identity ID (UUID)"
}
});
}
async function app() {
incode = initializeIncodeSDK();
identifyUser();
}
document.addEventListener("DOMContentLoaded", app);
2. Check for a face match between the user's selfie and an Incode identity.
renderLogin()
method response
renderLogin()
method response{
"customerId": "635f3a596a3dfd258aca0af3",
"token": "IDENTITY TOKEN",
"interviewId": "6350faae5d5aa3c7fa905cb9",
"interviewToken": "ONBOARDING SESSION TOKEN",
"transactionId": "64102af47b8072faffad19da",
"faceMatch": true,
"spoofAttempt": false,
"secondFactor": false,
"children": [],
"spoofConfidence": 0.00383246,
"recognitionConfidence": {
"confidence": 0.904334,
"candidates": []
},
"blurriness": 273.622,
"isBright": true,
"overallScore": 0.904334,
"overallStatus": "PASS",
"base64Image": ""
}
Let's explain some crucial properties from the response:
faceMatch
. Indicates if the user was successfully identified.customerId
. The Incode Identity ID.token
. The authentication token.interviewToken
. The session token for the user's original onboarding. You can use this value to access the origin of the user's onboarding session data in your application backend. For example, to get OCR data like name or address via the Omni API.transactionId
. The authentication attempt identifier.
3. Validate the authentication attempt using the/omni/authentication/verify
.
/omni/authentication/verify
.This step happens in backend:
The authentication attempt can only be verified in your application backend as the omni API requires and admin token.
curl --location '[YOUR INCODE API URL]/omni/' \
--header 'Accept: application/json' \
--header 'Content-Type: application/json' \
--header 'api-version: 1.0' \
--header 'x-api-key: INCODE API KEY' \
--header 'X-Incode-Hardware-Id: ADMIN TOKEN' \
--data '{
"transactionId": "AUTHENTICATION ATTEMPT IDENTIFIER FROM THE renderLogin() METHOD RESPONSE",
"token": "AUTHENTICATION TOKEN FROM THE renderLogin() METHOD RESPONSE",
"interviewToken": "The session token FROM THE renderLogin() METHOD RESPONSE"
}'
The /omni/authentication/verify
request body requires the transactionId
, token
and interviewToken
values from the renderLogin()
method response.
/omni/authentication/verify
response.
/omni/authentication/verify
response.{
"verified":true
}
{
"verified":true
"reason": 0
}
The verified
(boolean) key is the only value returned indicating the authentication is legitimate. As you can see in the diagram, you must validate this value to decide if the user continues with your application flow.
Updated 2 months ago
- You can download the code sample for this guide.
- Get a webhook notification every time an authentication is registered.