Redirect To Mobile
When verifying customers in a web app, it is best practice to redirect clients to their mobile phone. This is especially true if the app asks customers to submit any item in the list below.
- Selfie image
- ID images (front and back)
- Passport image
- Utility Bill image
- Bank Statement image
- Proof Of Address image
- Insurance Card image
The reason to redirect users to a mobile phone is because phones make it easy to take selfies and take pictures of documents. Additionally phone cameras are typically equipped with more capabilities to take better images then desktop cameras.
Enable Redirect To Mobile in Low Code Apps
In a low code apps, redirect to mobile is just a setting which must be enabled.
Using Workflows
Open a Workflow in edit mode -> Click pencil next to flow name -> Check the box to enable "Redirect to mobile".
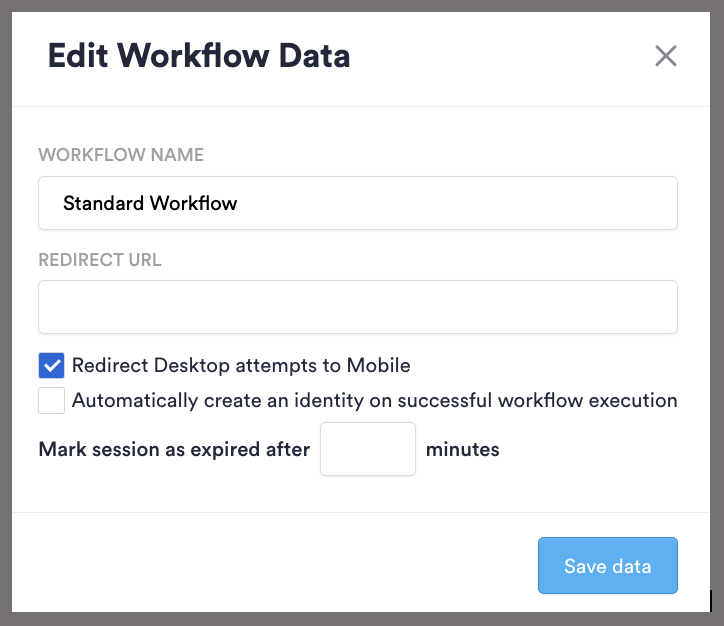
Workflows checkbox to redirect desktop users to their mobile phone.
Using Flows
Open a Flow in edit mode -> Click View Details -> Click Self Configuration -> Check the box to enable "Redirect to mobile".
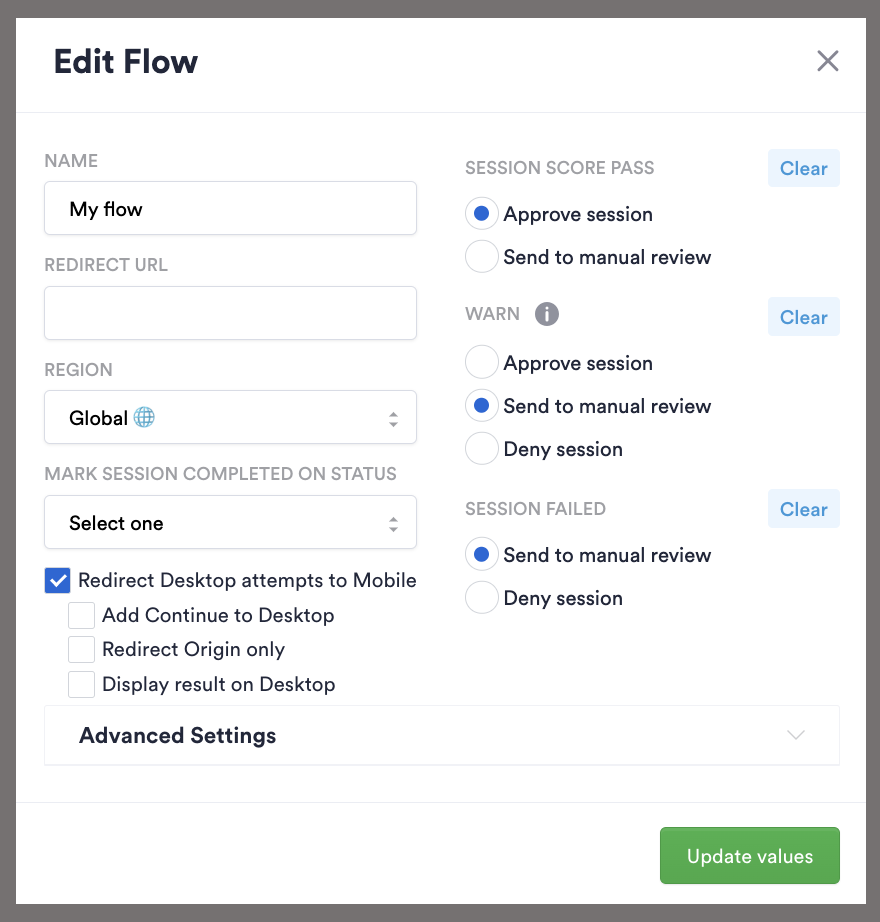
Flows checkbox to redirect desktop users to their mobile phone.
Enable Redirect To Mobile in Web SDK Apps
Apps developed with the SDK have the ability to redirect customers to their mobile devices. This is made possible by the renderRedirectToMobile() component. This component presents a QR code and a phone input field to the users, providing them with options to continue their onboarding session on their mobile devices
It is required to implement this only after initializing the SDK and obtaining a session token. This approach ensures a seamless transition and continuation of the user's onboarding process.
Note: Note the use of the isDesktop() condition. To make a smooth experience for users already on mobile devices, this is important.
Using renderRedirectToMobile()
const flowId = "your-flow-id";
const session = await onBoarding.createSession("ALL", null, {
configurationId: flowId,
});
if (onBoarding.isDesktop()) {
onBoarding.renderRedirectToMobile(containerRef.current, {
onSuccess: () => {
renderFinishScreen();
},
session: session,
flowId: flowId,
});
} else {
// show mobile normal flow
renderFrontId();
}
Managing Session on Redirect
One crucial aspect of integrating with the Incode Web SDK is the effective management of sessions during redirections.
Obtaining the UUID
After scanning a QR code or receiving an SMS message, a redirection to a predetermined URL will occur. This URL will carry a unique UUID parameter that must be retrieved to continue the session.
Here's a sample URL structure:
https://{{redirect-url}}/uuid={{uuid}}\
once on your app you will be able to retrieve the uuid using the available tools on your framework
// Obtaining the uuid in React
const queryParameters = new URLSearchParams(window.location.search)
const type = queryParameters.get("uuid")
Start a session with UUID
To maintain the session that started on the desktop, it's essential to replace the configurationId
parameter you'd typically use when starting a session with the received uuid
parameter. This substitution allows the user to progress seamlessly through the onboarding process.
Here's an example of how you can implement this:
await fetch(`${process.env.INCODE_API_URL}omni/start`, {
method: 'POST',
mode: 'cors',
cache: 'no-cache',
credentials: 'same-origin',
headers: {
'Access-Control-Allow-Origin': '*',
'Content-Type': 'application/json',
'api-version': "1.0",
'x-api-key': process.env.INCODE_API_KEY
},
redirect: 'follow',
referrerPolicy: 'no-referrer',
body: JSON.stringify({
countryCode: "ALL",
[params.uuid ? "uuid" : "configurationId"]: params.uuid || process.env.INCODE_CONFIG_ID
})
});
Updated 2 months ago