URL Redirect
In this approach you simply redirect the user to the flow, and then wait for the notification on a Webhook.
In this integration approach, you only care about redirecting your user to the onboarding URL and let them finish without the web app intervening again.
You know from the previous step that you need to Get an Onboarding URL, depending on your choice you have two options
A) Static URL
If you are using a Static URL just redirect the user to that URL with:
// Static URL: Copy the URL from the dashboard.
const url="url you copied from the dashboard.";
window.location.replace("https://url-you-copied-from-dashboard");
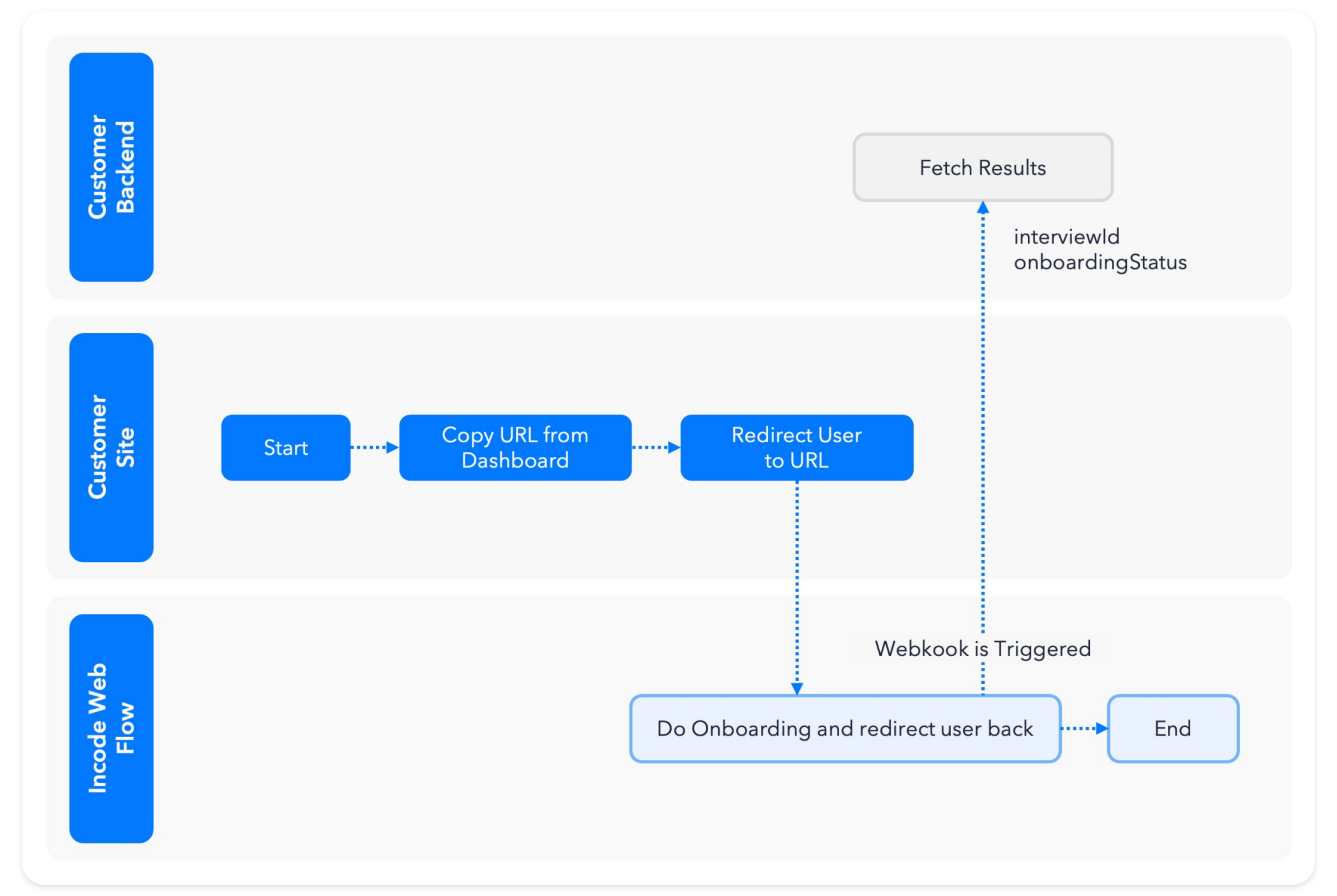
B) Dynamic URL
If you are using a Dynamic URL you will need to consume the url from the backend that was created in the previous step.
// Dynamic URL: Call the backend to generate it and in
// the frontend and just consume the url
const response = await fetch(`https://you.backend.app/onboarding-url`, {});
const { url } = await response.json();
// Redirect user to incode workflow
window.location.replace(url);
When using this integration your only option to fetch the scores is waiting for the Webhook notification to arrive into your backend, you can learn how to do that in: Fetch Results
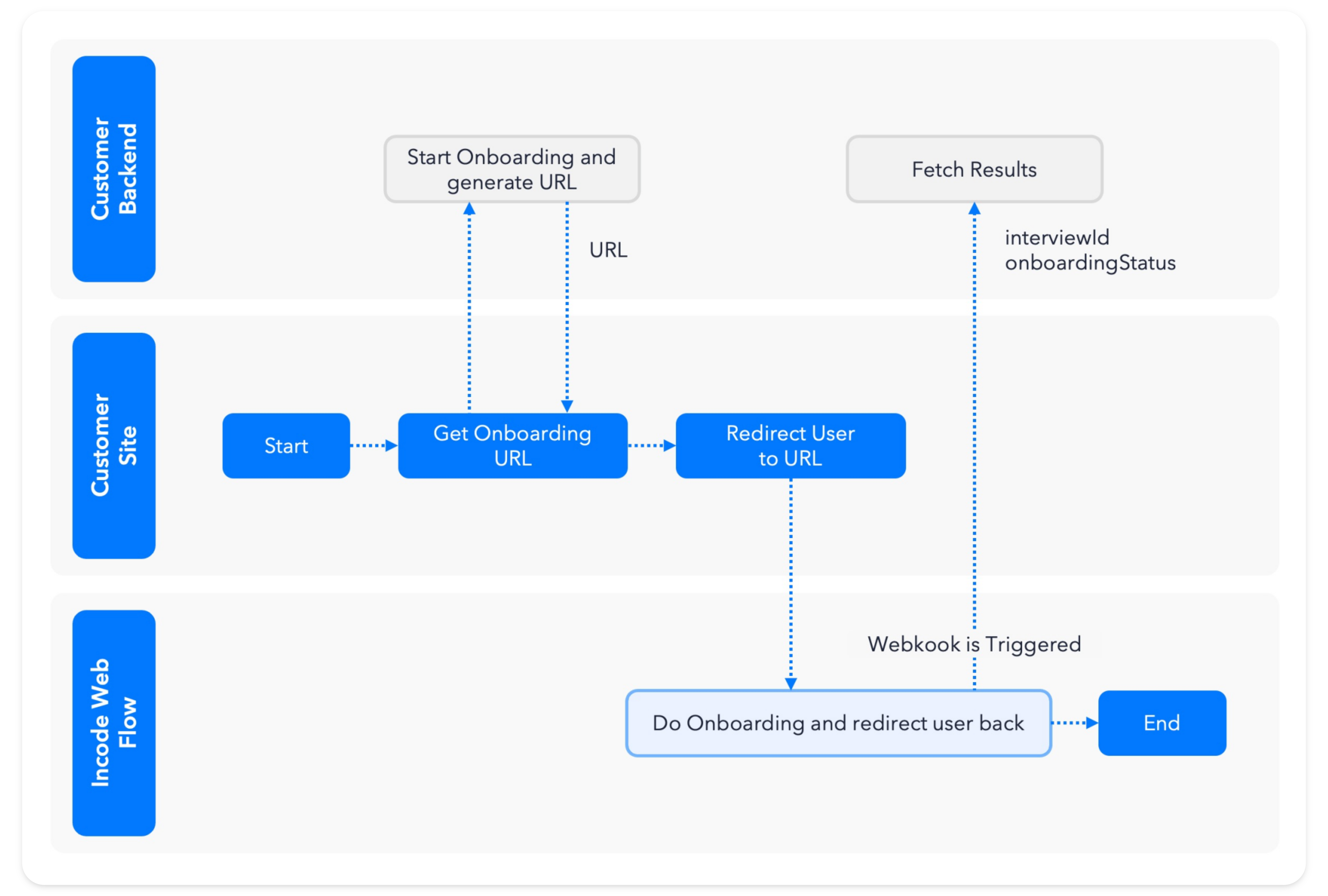
Updated about 1 month ago