Redirect and Back
In this approach you redirect your user to the onboarding url, and then back to complete the process.
This integration approach has the following steps:
- Get an Onboarding URL
- Set some reference about the session (correlationId)
- Redirect the user to that URL.
- The user will do onboarding and be redirected back to the application.
- Recover the correlationId you set up in step 2.
- Fetch Results using that correlationId
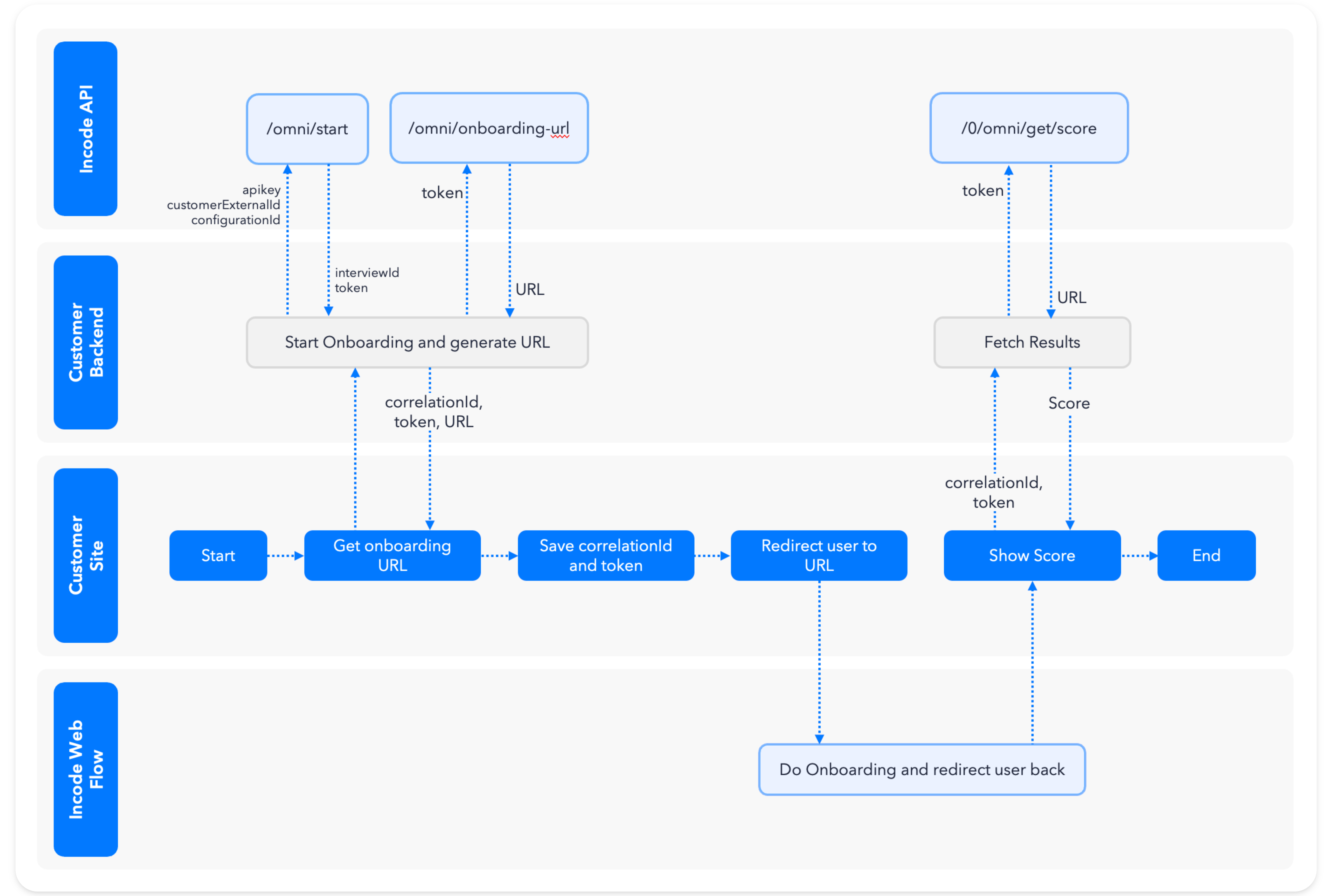
1. Get onboarding URL
Follow the steps on Get Onboarding URL to set up the backend URL creation, and then set up an HTML file (probably index.html
) with its corresponding javascript file.
Call the backend using fetch
and extract the data needed for the next steps.
// Dynamic URL: Call the backend to generate it and in
// the frontend and just consume the URL
const response = await fetch(`https://you.backend.app/onboarding-url`,{});
const {url, token, interviewId} = await response.json();
2. Set some references about the session
You need to carry a correlationId
in some way, for this example we will use interviewId
as the correlationId
, simply store it in LocalStorage along with the token
localStorage.setItem("interviewId", interviewId);localStorage.getItem('interviewId');
localStorage.setItem("token", token);
3. Redirect the user to the URL
window.location.replace(url);
4. Receive the user back
For the user to come back you need to set up the redirectURL in Get Onboarding URL, independently of the method you choose, you need to create the endpoint where the user is coming back.
Create an HTML file named finished.html
with its corresponding javascript file.
5. Retrieve the correlationId and Token
If you followed these steps, you can then retrieve the correlation (in this case interviewId
) and token
from localStorage.
const interviewId = localStorage.getItem('interviewId');
const token = localStorage.getItem('token');
6. Fetch the scores
Fetching the scores happens in the backend, so let's close this step by asking the data from the backend, you can see exactly what the scores mean and what other things you can fetch in the next section.
async function fetchScore(interviewId, token) {
const response = await fetch(
`<url-of-your-backend>/fetch-score=?interviewId=${interviewId}`,
{ "x-token": token }
);
const {status} = await response.json();
return {status}
}
// 5.- Retrieve the correlationId and Token
const interviewId = localStorage.getItem('interviewId')
const token = localStorage.getItem('token');;
// 6.- Fetch score
const response = await fetch(
`<url-of-your-backend>/fetch-score=?interviewId=${interviewId}`,
{ "x-token": token }
);
const {status} = await response.json();
if(status==='OK'){
console.log('You sucessfully onboarded');
} else {
console.log('You didn\'t passed');
}
On Security
While scores could be retrieved in backend using an Admin Token, requiring a regular Session Token keeps this endpoint open to only the current session.
A step further into securing this would be to store the token in the backend behind a cookie or in the database, limiting even more what a malicious actor can do.
Updated about 18 hours ago