Embedded Iframe
In this approach you show the flow inside an Iframe while you poll for the onboarding status.
This integration approach has the following steps:
- Get an Onboarding URL
- Create an Iframe with the URL
- The user will do onboarding inside the Iframe; meanwhile, you poll the backend for the
ONBOARDING_FINISHED
status. - As soon as the onboarding finishes, close the Iframe.
- Fetch Results
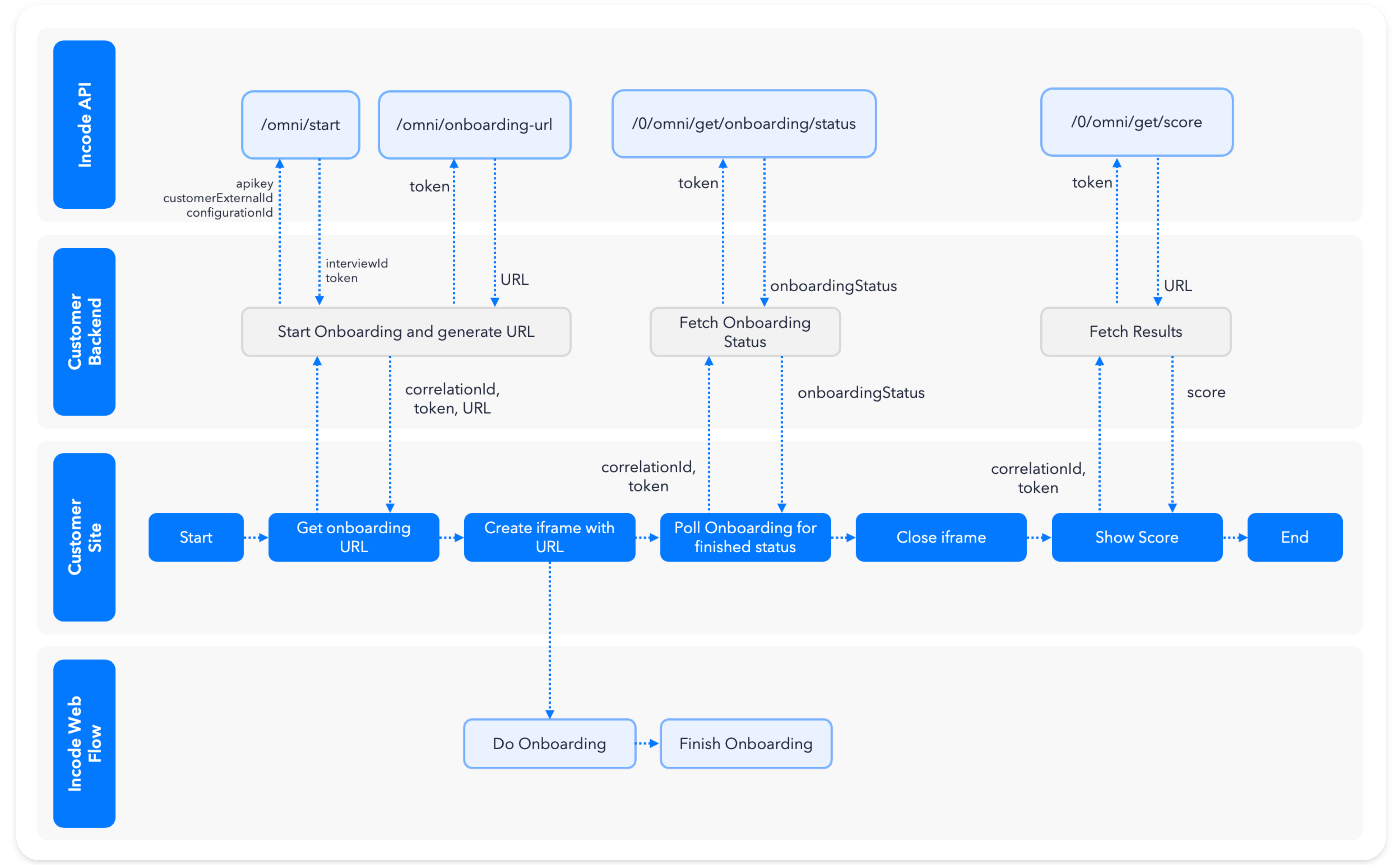
1. Get onboarding URL
Follow the steps on Get Onboarding URL to set up the backend URL creation, and then set up an HTML file (probably index.html
) with its corresponding javascript file.
Call the backend using fetch
and extract the data needed for the next steps.
// Dynamic URL: Call the backend to generate it and in
// the frontend and just consume the URL
const response = await fetch(`https://you.backend.app/onboarding-url`,{});
const {url, token, interviewId} = await response.json();
correlationId
can be anything, in this case, we are using interviewId
.
2. Create an Iframe with the URL
To work correctly, the Iframe created needs to comply with several conditions: set it to 100% and allow the usage of geolocation, microphone, and camera. Also, for our onboarding functionality process, a gyroscope and accelerometer are needed for features with motion, orientation, and gesture-based interactions.
Set its URL, and the user can do their onboarding.
const createIframe = (url) => {
/* Get the container where we are going to insert the iframe */
const app = document.getElementById('app'); //reference to a element on index.html page
/* Create the iframe */
const frame = document.createElement('iframe');
/* Set the URL */
frame.src = url;
frame.id = 'app-iframe'
frame.width = '100%';
frame.height = '100%';
/* Allow the iframe to use the camera and other media */
frame.allowUserMedia = true;
frame.setAttribute('frameborder', '0');
/* Allow specific permissions. Notice the wildcard character (*) */
frame.setAttribute('allow', 'geolocation *; microphone *; camera *; accelerometer *; gyroscope *;');
/* Insert iframe into container */
app.appendChild(frame);
}
createIframe(url);
3. Poll for the onboarding status
There is no way to directly monitor the progress of an Iframe, so instead we will poll the API for the onboarding status. Full list can be found in Onboarding Statuses, we are waiting for the ONBOARDING_FINISHED
one.
async function fetchOnboardingStatus(interviewId) {
const response = await fetch(
`<url-of-your-backend>/onboarding-status?interviewId=${interviewId}`,
{ "x-token": token }
);
const {onboardingStatus} = await response.json();
return {onboardingStatus}
}
const interval = setInterval(async () => {
const onboarding = await fetchOnboardingStatus(interviewId);
if (onboarding.onboardingStatus==='ONBOARDING_FINISHED'){
clearInterval(interval);
// Remove iframe from the parent node
//const frame = document.getElementById('app-iframe');
//frame.parentNode.removeChild(frame);
// Here You are ready to fetch scores
}
}, 1000);
4. Close Iframe
Closing an Iframe might be tricky. For it to really stop, you need to do it by referencing its parent node.
// Remove iframe from the parent node
const frame = document.getElementById('app-iframe');
frame.parentNode.removeChild(frame);
5. Fetch the scores
Fetching the scores happens in the backend, so let's close this step by asking the data from the backend, you can see exactly what the scores mean and what other things you can fetch in the next section.
async function fetchScore(interviewId, token) {
const response = await fetch(
`<url-of-your-backend>/fetch-score?interviewId=${interviewId}`,
{ "x-token": token }
);
const {status} = await response.json();
return {status}
}
// 5.- Retrieve the correlationId and Token
const interviewId = localStorage.getItem('interviewId');
const token ItelocalStorage.getItem('token');
// 6.- Fetch score
const { status } = await fetchScore(interviewId, token);
if(status==='OK'){
console.log('You sucessfully onboarded');
} else {
console.log('You didn\'t passed');
}
On Security
While scores and onboarding status could be retrieved in backend using an Admin Token, requiring a regular Session Token keeps this endpoint open to only the current session.
A step further into securing this would be to store the token in the backend behind a cookie or in the database, limiting even more what a malicious actor can do.
Updated about 20 hours ago